This assignment will incorporate most structures that you learned in our
class. You will use classes and pointers to implement this TIC-TAC-TOE
program.
You will set up a table such as the figure below. (NO NEED to create
Graphics, but 15 extras points will be given to those that implement this
program using graphics like the figure bellow.
The player will play against the computer!
There is a java
version of this game if you need to familiarize yourself with this game.
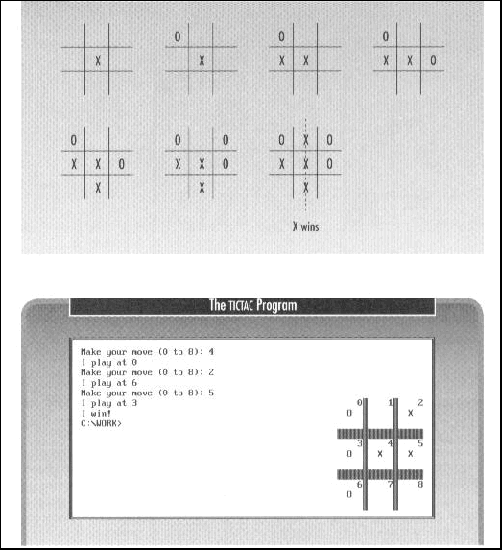
The basic structure for this program is as follow, although you can modify
it, but remember to use classes and pointers.
//
tictac.cpp
//
tic-tac-toe game
#include
<iostream.h>
#include <conio
h> // for
gotoxy()
#include <process.h>
// for exit()
enum
whoplays { human, machine };
enum cell { empty, full };
enum boolean { false, true };
int
deep = O; // recursion depth
class
Position // represents one board position
{
private:
cell
mcell[9];
cell hcell[9];
whoplays player;
public:
Position();
// constructor
boolean IsWin();
// win in this position?
boolean IsLegal(int);
// move is legal?
void MakeMove(int);
// make move
void SetPlayer(whoplays); // swap player and machine
static void InitDisplay();// displays lines & numbers
void Display();
// display board position
int Evaluate(int&);
// score this position
};